Oracle Service Bus: WLST Fundamentals
Published on: Author: Michel Schildmeijer Category: OracleIn my series of blogs about the Oracle Service Bus and WLST scripting I’d like to focus some more on the fundamentals, which will guide you through building smart generic scripts for your OSB/WebLogic domain. As you might know, WebLogic uses the WLST build on Python and Jython for getting tasks programmatically standardized and, if necessary, automated. Now, before you start using WLST together with Oracle Service Bus, it may be useful to understand some fundamentals.
Just as the MBeans in a standard WebLogic Domain, Oracle Service Bus uses its own MBeans on top of it. You can use this to request specific information about the configuration, resources such as proxy and business services. You also can export and import a certain configuration like you do in the Service Bus Console.
When you want to create a WLST script for some purpose, the following MBeans are the most important to start with:
- SessionManagementBean: Used to create, save or discard a session, the same way as in the console. For doing this you can use the following methods:
- Creates the session ( can also be multiple )
- Commit your changes
- Rollback your changes before activate
- Exit when actions are done or rollbacked.
createSession() activateSession() discardSession() sessionExists()
These actions you normally do in the console using these buttons:
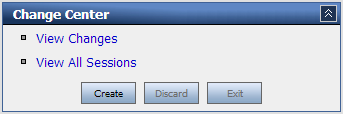
- ALSBConfgurationMBean: For the import and export of OSB configurations, updating specific information such as endpoint URI’s, and investigate configurations and resources. With this MBean you can manage your OSB configuration, which, packaged in a JAR file, can be Proxy and BusinessServices, WSDL’s or other resources.
- ServiceDomainMBean: This MBean finds any service enabled for monitoring, and get or reset statistics for services which are enabled for monitoring.
When you start WLST, standard WebLogic modules are loaded but specific OSB you should import in your script to use are:
from com.bea.wli.sb.management.configuration import ALSBConfigurationMBean from com.bea.wli.monitoring import ServiceDomainMBean
to be found in com.bea.common.configfwk_1.5.0.0.jar, sb-kernel-api.jar and sb-kernel-impl.jar in your OSB library home.
Creating the session:
sessionManager = findService(SessionManagementMBean.NAME, SessionManagementMBean.TYPE) sessionManager.createSession(sessionName)
Then do some stuff….
And save the session or discard when something went wrong:
# Activate the change session print 'Activating my session...' sessionManager.activateSession(sessionName, 'Doing stuff session activated') except: if sessionManager != None: sessionManager.discardSession(sessionName) raise
Use of resource references
For the identification of projects, folders and other resources you can use the Ref() class, and you will use it with the ALSBConfgurationMBean. You can create, delete or export projects and folders with the following methods:
createProject(Ref project, String Description) createFolder(Ref folder, String Description) delete() importUploaded(ALSBImportPlan plan) to implement a customization plan Customize(List customizations) ExportProjects(Collection Refs, String ….
Normally it’s done through this section in the sbconsole:
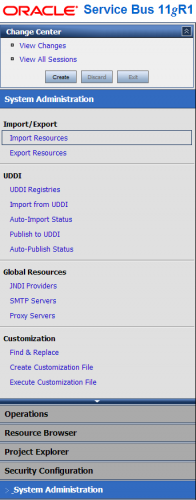
Below you see a snippet of how to import a Customization (with the customization stored in an XML file):
from com.bea.wli.sb.management.configuration import SessionManagementMBean from com.bea.wli.sb.management.configuration import ALSBConfigurationMBean from com.bea.wli.config import Ref .. sessionName = createSessionName() print 'Created session', sessionName SessionMBean = getSessionMBean(sessionName) print 'SessionMBean started session' ALSBConfigurationMBean = findService(String("ALSBConfiguration.").concat(sessionName), "com.bea.wli.sb.management.configuration.ALSBConfigurationMBean") print "ALSBConfiguration MBean found", ALSBConfigurationMBean #customize if a customization file is specified #affects only the created resources if customFile != None : print 'Loading customization File', customFile iStream = FileInputStream(customFile) customizationList = Customization.fromXML(iStream) for customization in customizationList: print '\n customization', customization.getDescription() print customizationList.size() ALSBConfigurationMBean.customize(customizationList) print 'Customization applied' SessionMBean.commitSession(sessionName) print 'session committed' except: print "Unexpected error:", sys.exc_info()[0] if SessionMBean != None: SessionMBean.discardSession(sessionName) raise
… and so on.
ServiceDomainMBean
Through this interface you can get loads of statistics of your services. Some of the methods to use are:
- Determining which services are enabled for monitoring
getMonitoredBusinessServiceRefs() getMonitoredProxyServiceRefs
- Gives back runtime statistics collected. You can specify a server name, or get back cluster wide statistics from an aggregated view of statistics of all managed servers).
getBusinessServiceStatistics() getProxyServiceStatistics()
A short sample below:
stats = cmo.getProxyServiceStatistics([ref],ResourceType.SERVICE.value(),'') for ps in stats[ref].getAllResourceStatistics(): for s in ps.getStatistics(): if s.getType() == StatisticType.COUNT: print s.getName() + "("+ str(s.getType()) +"): " + str(s.getCount()) if s.getType() == StatisticType.INTERVAL: print ( s.getName() + "("+ str(s.getType()) +"): " + str(s.getMin()) + " " + str(s.getMax()) + " " + str(s.getAverage()) + " " + str(s.getSum()) if s.getType() == StatisticType.STATUS: print s.getName() + "("+ str(s.getType()) +"): " + str(s.getCurrentStatus()) + "(" + str(s.getInitialStatus()) + ")"
Now, these are some basics to help you build your own customized and nifty scripts, have fun and good luck with it!
Very good article. I need help on creating JNDI Providers using WLST. Can you please give me sample script to do that.? Thanks in advanced